Generate Key In Object If Does Not Have When Mapping
- Generate Key In Object If Does Not Have When Mapping Meaning
- Generate Key In Object If Does Not Have When Mapping Mean
- Hibernate Tutorial
Then regarding your views which don't have any columns to make a row unique, the easiest way to guarantee Entity Framework can map each of your view's row to their own objects is to create a separate column for your view's primary key, a good candidate is to just create a row number column on each row. A Set is a java collection that does not contain any duplicate element. More formally, sets contain no pair of elements e1 and e2 such that e1.equals(e2), and at most, one null element. So, objects to be added to a set must implement both the equals and hashCode methods so that Java can determine whether any two elements/objects are identical.
- Hibernate Useful Resources
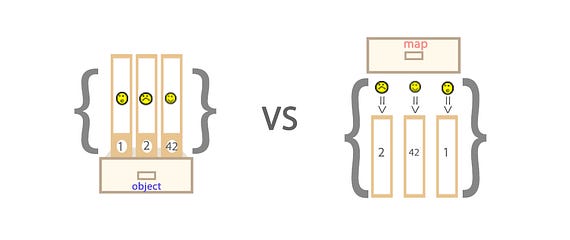
- Selected Reading
A Many-to-Many mapping can be implemented using a Set java collection that does not contain any duplicate element. We already have seen how to map Set collection in hibernate, so if you already learned Set mapping, then you are all set to go with manyto-many mapping.
A Set is mapped with a <set> element in the mapping table and initialized with java.util.HashSet. You can use Set collection in your class when there is no duplicate element required in the collection.
Define RDBMS Tables
Consider a situation where we need to store our employee records in EMPLOYEE table, which will have the following structure −
Further, assume each employee can have one or more certificate associated with him/her and a similar certificate can be associated with more than one employee. We will store certificate related information in a separate table, which has the following structure −
Now to implement many-to-many relationship between EMPLOYEE and CERTIFICATE objects, we would have to introduce one more intermediate table having Employee ID and Certificate ID as follows −
Define POJO Classes
Let us implement our POJO class Employee, which will be used to persist the objects related to EMPLOYEE table and having a collection of certificates in Set variable.
Now let us define another POJO class corresponding to CERTIFICATE table so that certificate objects can be stored and retrieved into the CERTIFICATE table. This class should also implement both the equals() and hashCode() methods so that Java can determine whether any two elements/objects are identical.
Define Hibernate Mapping File
Let us develop our mapping file, which instructs Hibernate — how to map the defined classes to the database tables. The <set> element will be used to define the rule for manyto-many relationship.
You should save the mapping document in a file with the format <classname>.hbm.xml. We saved our mapping document in the file Employee.hbm.xml. You are already familiar with most of the mapping detail, but let us see all the elements of mapping file once again −
The mapping document is an XML document having <hibernate-mapping> as the root element which contains two <class> elements corresponding to each class.
The <class> elements are used to define specific mappings from a Java classes to the database tables. The Java class name is specified using the name attribute of the class element and the database table name is specified using the table attribute.
The <meta> element is optional element and can be used to create the class description.
The <id> element maps the unique ID attribute in class to the primary key of the database table. The name attribute of the id element refers to the property in the class and the column attribute refers to the column in the database table. The type attribute holds the hibernate mapping type, this mapping types will convert from Java to SQL data type.
The <generator> element within the id element is used to generate the primary key values automatically. The class attribute of the generator element is set to native to let hibernate pick up either identity, sequence or hilo algorithm to create primary key depending upon the capabilities of the underlying database.
The <property> element is used to map a Java class property to a column in the database table. The name attribute of the element refers to the property in the class and the column attribute refers to the column in the database table. The type attribute holds the hibernate mapping type, this mapping types will convert from Java to SQL data type.
The <set> element sets the relationship between Certificate and Employee classes. We set cascade attribute to save-update to tell Hibernate to persist the Certificate objects for SAVE i.e. CREATE and UPDATE operations at the same time as the Employee objects. The name attribute is set to the defined Set variable in the parent class, in our case it is certificates. For each set variable, we need to define a separate set element in the mapping file. Here we used name attribute to set the intermediate table name to EMP_CERT.
This release was created for you, eager to use Microsoft Office 2011 MAC full and with without limitations.Our intentions are not to harm Microsoft software company but to give the possibility to those who can not pay for any pieceof software out there. Our releases are to prove that we can! Ms office 2011 key generator. This should be your intention too, as a user, to fully evaluate Microsoft Office 2011 MAC withoutrestrictions and then decide.If you are keeping the software and want to use it longer than its trial time, we strongly encourage you purchasing the license keyfrom Microsoft official website.
The <key> element is the column in the EMP_CERT table that holds the foreign key to the parent object ie. table EMPLOYEE and links to the certification_id in the CERTIFICATE table.
The <many-to-many> element indicates that one Employee object relates to many Certificate objects and column attributes are used to link intermediate EMP_CERT.
Create Application Class
Finally, we will create our application class with the main() method to run the application. We will use this application to save few Employee's records along with their certificates and then we will apply CRUD operations on those records.
Compilation and Execution
Here are the steps to compile and run the above mentioned application. Make sure you have set PATH and CLASSPATH appropriately before proceeding for the compilation and execution.
Create hibernate.cfg.xml configuration file as explained in configuration chapter.
Create Employee.hbm.xml mapping file as shown above.
Create Employee.java source file as shown above and compile it.
Create Certificate.java source file as shown above and compile it.
Create ManageEmployee.java source file as shown above and compile it.
Execute ManageEmployee binary to run the program.
You would get the following result on the screen, and same time records would be created in EMPLOYEE, EMP_CERT and CERTIFICATE tables.
If you check your EMPLOYEE, EMP_CERT and CERTIFICATE tables, they should have following records −
-->Public Key Cryptography is a form of message secrecy in which a user creates a public key and a private key. The private key is kept secret, whereas the public key can be distributed to others. Although the keys are mathematically related, the private key cannot be easily derived by using the public key. The public key can be used to encrypt data which only the corresponding private key will be able to decrypt. This can be used for encrypting messages to the owner of the private key. Similarly the owner of a private key can encrypt data which can only be decrypted with the public key. This use forms the basis of digital certificates in which information contained in the certificate is encrypted by the owner of a private key, assuring the author of the contents. Since the encrypting and decrypting keys are different they are known as asymmetric keys.
Certificates and asymmetric keys are both ways to use asymmetric encryption. Certificates are often used as containers for asymmetric keys because they can contain more information such as expiry dates and issuers. There is no difference between the two mechanisms for the cryptographic algorithm, and no difference in strength given the same key length. Generally, you use a certificate to encrypt other types of encryption keys in a database, or to sign code modules.
Certificates and asymmetric keys can decrypt data that the other encrypts. Generally, you use asymmetric encryption to encrypt a symmetric key for storage in a database.
A public key does not have a particular format like a certificate would have, and you cannot export it to a file.

Note
SQL Server contains features that enable you to create and manage certificates and keys for use with the server and database. SQL Server cannot be used to create and manage certificates and keys with other applications or in the operating system.
Certificates
A certificate is a digitally signed security object that contains a public (and optionally a private) key for SQL Server. You can use externally generated certificates or SQL Server can generate certificates.
Note
Generate Key In Object If Does Not Have When Mapping Meaning
SQL Server certificates comply with the IETF X.509v3 certificate standard.
Certificates are useful because of the option of both exporting and importing keys to X.509 certificate files. The syntax for creating certificates allows for creation options for certificates such as an expiry date.
Using a Certificate in SQL Server
Certificates can be used to help secure connections, in database mirroring, to sign packages and other objects, or to encrypt data or connections. The following table lists additional resources for certificates in SQL Server.
Topic | Description |
---|---|
CREATE CERTIFICATE (Transact-SQL) | Explains the command for creating certificates. |
Identify the Source of Packages with Digital Signatures | Shows information about how to use certificates to sign software packages. |
Use Certificates for a Database Mirroring Endpoint (Transact-SQL) | Covers information about how to use certificates with Database Mirroring. |
Asymmetric Keys
Asymmetric keys are used for securing symmetric keys. They can also be used for limited data encryption and to digitally sign database objects. An asymmetric key consists of a private key and a corresponding public key. For more information about asymmetric keys, see CREATE ASYMMETRIC KEY (Transact-SQL).
Asymmetric keys can be imported from strong name key files, but they cannot be exported. They also do not have expiry options. Asymmetric keys cannot encrypt connections.
Using an Asymmetric Key in SQL Server
Asymmetric keys can be used to help secure data or sign plaintext. The following table lists additional resources for asymmetric keys in SQL Server.
Topic | Description |
---|---|
CREATE ASYMMETRIC KEY (Transact-SQL) | Explains the command for creating asymmetric keys. |
SIGNBYASYMKEY (Transact-SQL) | Displays the options for signing objects. |
Tools
Microsoft provides tools and utilities that will generate certificates and strong name key files. These tools offer a richer amount of flexibility in the key generation process than the SQL Server syntax. You can use these tools to create RSA keys with more complex key lengths and then import them into SQL Server. The following table explains shows where to find these tools.
Tool | Purpose |
makecert | Creates certificates. |
sn | Creates strong names for symmetric keys. |
Related Tasks
See Also
Generate Key In Object If Does Not Have When Mapping Mean
sys.certificates (Transact-SQL)
Transparent Data Encryption (TDE)